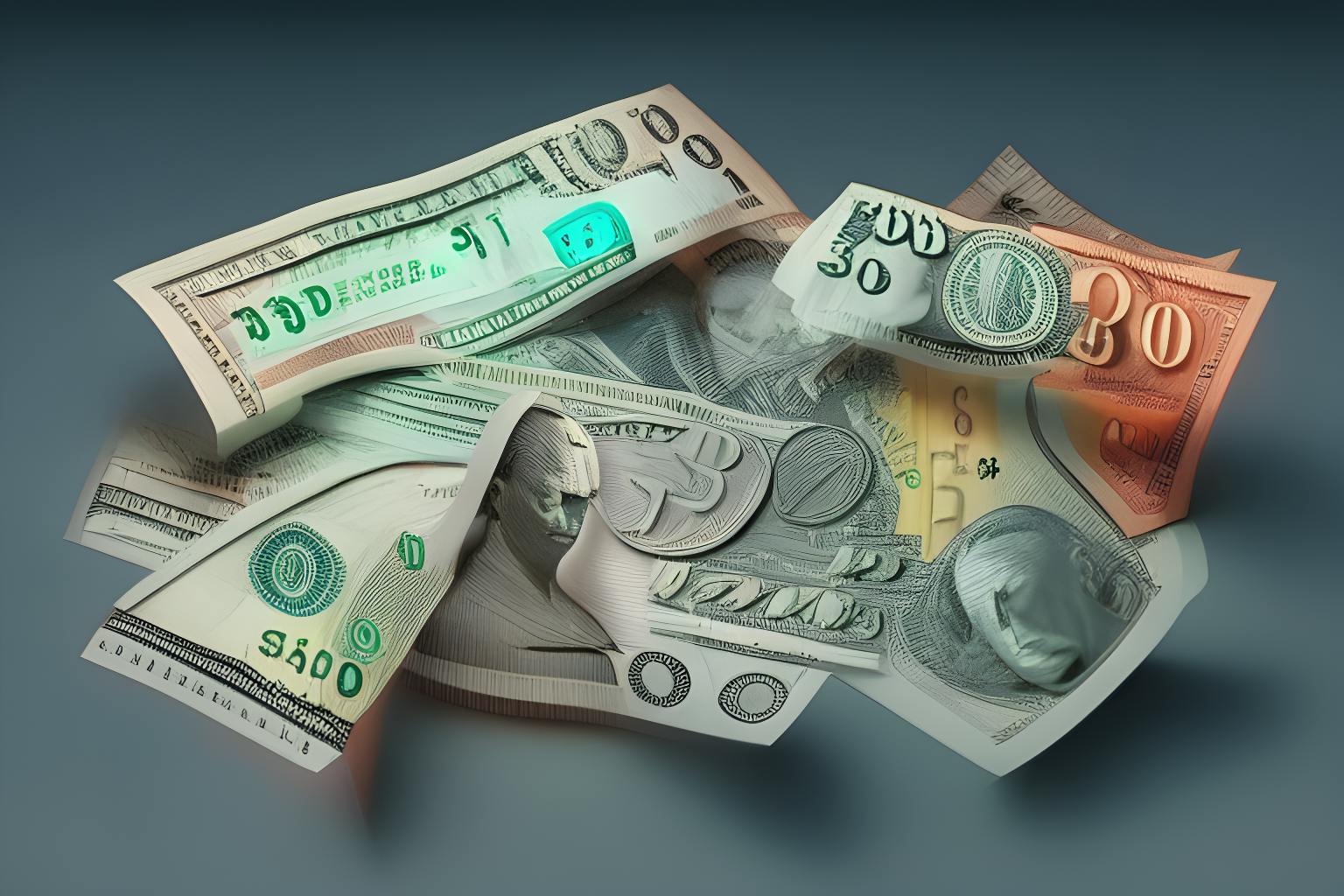
Создание конвертера валют во Flutter
23 июня 2023 г.В этой статье мы будем создавать приложение для конвертации валют на Flutter. Вы можете найти видео на YouTube в конце статьи с моим пошаговым процессом.
Давайте погрузимся прямо в код.
main.dart
import 'package:flutter/material.dart';
import 'package:testapp/dollar_to_inr.dart';
import 'package:testapp/inr_to_dollar.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
home: CurrencyConverter(),
);
}
}
class CurrencyConverter extends StatelessWidget {
const CurrencyConverter({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Currency Converter'),
),
body: Center(
child: Column(
//for the buttons to be in center
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () {
//on pressing dollar to INr we should navigate
//to the dollar_to_inr widget
Navigator.of(context).push(
MaterialPageRoute(builder: (context) => DollarToINR()));
},
child: Text('Dollar To INR'),
),
//create sized box for space between
//two buttons
const SizedBox(
height: 30,
),
//another elevated button for inr to dollar
ElevatedButton(
onPressed: () {
//on pressing this button
//we should naviaget to inr_to_dollar.dart
//widget
Navigator.of(context).push(
MaterialPageRoute(builder: (context) => InrToDollar()));
},
child: Text(
'INR to Dollar',
),
)
],
),
),
);
}
}
inr_to_dollar.dart
import 'package:flutter/material.dart';
import 'package:flutter/src/widgets/framework.dart';
import 'package:flutter/src/widgets/placeholder.dart';
class InrToDollar extends StatefulWidget {
const InrToDollar({super.key});
@override
State<InrToDollar> createState() => _InrToDollarState();
}
class _InrToDollarState extends State<InrToDollar> {
//create controller for inr
TextEditingController inrController = TextEditingController();
//creating the varibale to store the dollar value
double dollar = 0.0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Inr to Dollar Converter'),
),
body: Container(
//give some padding
padding: EdgeInsets.all(20),
//here we need to create
//one text filed to take input form user
//and one button
//so we will use here column widget
child: Column(
children: [
TextField(
controller: inrController,
//some box around text field
decoration: const InputDecoration(
border: OutlineInputBorder(),
//labele text
labelText: 'Enter Inr',
),
),
//need some space between
//textfield and button
const SizedBox(
height: 30,
),
//create convert button
ElevatedButton(
onPressed: () {
//on pressing the convert we
//should convert the inr to dollar
setState(() {
dollar = double.parse(inrController.text) * 0.012;
});
},
child: Text("Convert"),
),
//for some space between button and text
SizedBox(
height: 30,
),
//now we have to show dollar on screen
//so we will use here text widget
Text(
"Dollar : " + dollar.toString(),
//increse some font size
style: TextStyle(
fontSize: 30,
),
),
],
),
),
);
}
}
Dollar_to_inr.dart
import 'package:flutter/src/widgets/framework.dart';
import 'package:flutter/src/widgets/placeholder.dart';
import 'package:flutter/material.dart';
class DollarToINR extends StatefulWidget {
const DollarToINR({super.key});
@override
State<DollarToINR> createState() => _DollarToINRState();
}
class _DollarToINRState extends State<DollarToINR> {
//create controller for dollar
TextEditingController dollarController = TextEditingController();
//creating the varibale to store the inr value
double inr = 0.0;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Dollar to inr Converter'),
),
body: Container(
//give some padding
padding: EdgeInsets.all(20),
//here we need to create
//one text filed to take input form user
//and one button
//so we will use here column widget
child: Column(
children: [
TextField(
controller: dollarController,
//some box around text field
decoration: const InputDecoration(
border: OutlineInputBorder(),
//labele text
labelText: 'Enter Dollar',
),
),
//need some space between
//textfield and button
const SizedBox(
height: 30,
),
//create convert button
ElevatedButton(
onPressed: () {
//on pressing the convert we
//should convert the inr to dollar
setState(() {
inr = double.parse(dollarController.text) * 82.40;
});
},
child: Text("Convert"),
),
//for some space between button and text
SizedBox(
height: 30,
),
//now we have to show dollar on screen
//so we will use here text widget
Text(
"Inr : " + inr.toString(),
//increse some font size
style: TextStyle(
fontSize: 30,
),
),
],
),
),
);
}
}
Пошаговый процесс
https://youtu.be/705GO3kj9FU?embedable=true
:::информация Также опубликовано здесь
Главное изображение для этой статьи было создано HackerNoon's AI Image Generator с помощью подсказки "конвертер валют".
:::
Оригинал